In our last article, we saw that it was possible to use CDS Simple Types and CDS Enumerated Types within CDS Table Entities. These types can also be used for other purposes, which we’ll now explore.
All code is available in our Gitlab.
1. CDS Simple Types
- CDS SImple Types are used to type the elements or parameters of a CDS.
To demonstrate this case, we’ll take the example of the bakery created in our last article. And mainly this CDS:
@ClientHandling.type: #CLIENT_DEPENDENT
@AbapCatalog.deliveryClass: #APPLICATION_DATA
@AccessControl.authorizationCheck: #NOT_REQUIRED
@EndUserText.label: 'Bakery Orders'
define root table entity ZI_BAKERY_ORDERS
{
key uuid : uuid;
deliveryAddress : abap.char( 100 );
orderDate : abap.datn;
//every order is linked to (at least) one or many items
_bakeryOrdersItems : composition of exact one to many ZI_BAKERY_ORDERS_ITEMS;
}
Here, we can see that we’re using the abap.char( 100 ) type to define the address, but this isn’t very meaningful. Also, as the field description is based on the data Element, we’ll need to add a manual annotation in the CDS of type @EndUserText.label, to define the display on the UI (in Fiori, for example), as no label is defined on type abap.char.
So we’re going to replace abap.char with a CDS Simple Type that we’ll create.
To do this, search in Core Data Services: “Types”.
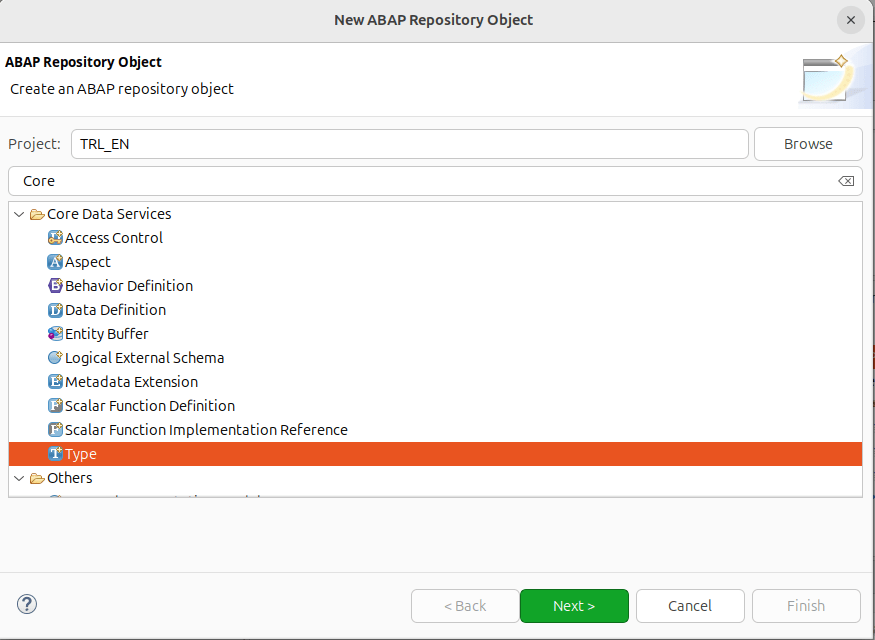
Then give it a name and a description. Next, we can assign an elementary data type (here still abap.char) to our ZCUSTOMER_ADDRESS type.
We also define labels to make them easier for the user to understand when they are displayed.
@EndUserText.label: 'Customer Address' // Human-readable text to be displayed on UIs
@EndUserText.quickInfo: 'Delivery Address of the customer' // Provides additional information compared to the label text.
@EndUserText.heading: 'Customer Address' //Human-readable text that is displayed as column headers
define type ZCUSTOMER_ADDRESS: abap.char( 100 )
Then we can use this Type in our CDS :
@ClientHandling.type: #CLIENT_DEPENDENT
@AbapCatalog.deliveryClass: #APPLICATION_DATA
@AccessControl.authorizationCheck: #NOT_REQUIRED
@EndUserText.label: 'Bakery Orders'
define root table entity ZI_BAKERY_ORDERS
{
key uuid : uuid;
deliveryAddress : ZCUSTOMER_ADDRESS;
orderDate : abap.datn;
//every order is linked to (at least) one or many items
_bakeryOrdersItems : composition of exact one to many ZI_BAKERY_ORDERS_ITEMS;
}
- Next, it’s important to note that a CDS Simple Type can be inherited from another CDS Simple Type.
In this case, the new Simple Type CDS retains all the technical characteristics, as well as the annotations, except that the annotations can be replaced if we wish.
This makes it possible, for example, to define a “basic” CDS Simple Type , which would technically define the Type, and which would then be used by other CDS Simple Type sharing the same technical characteristics. In this way, if a change is required ( for example, from abap.char( 100 ), to abap.char( 150 ) ), it need only be made in one place.
For example, here we can inherit the CDS Simple Type ZCUSTOMER_ADDRESS and use it to define other CDS Simple Types that share the same technical base.
ZPASTRY_CUSTOMER_ADDRESS for bakery customer addresses
@EndUserText.label: 'Pastry Customer Address' // Human-readable text to be displayed on UIs
@EndUserText.quickInfo: 'Delivery Address of the pastry customer' // Provides additional information compared to the label text.
@EndUserText.heading: 'Pastry Customer Address' //Human-readable text that is displayed as column headers
define type ZPASTRY_CUSTOMER_ADDRESS: ZCUSTOMER_ADDRESS //abap.char( 100 )
And like this, we can replace the annotations to make the label more precise, but we keep the technical characteristics linked to the ZCUSTOMER_ADDRESS type.
The final result is :
@ClientHandling.type: #CLIENT_DEPENDENT
@AbapCatalog.deliveryClass: #APPLICATION_DATA
@AccessControl.authorizationCheck: #NOT_REQUIRED
@EndUserText.label: 'Bakery Orders'
define root table entity ZI_BAKERY_ORDERS
{
key uuid : uuid;
deliveryAddress : ZPASTRY_CUSTOMER_ADDRESS;
orderDate : abap.datn;
//every order is linked to (at least) one or many items
_bakeryOrdersItems : composition of exact one to many ZI_BAKERY_ORDERS_ITEMS;
}
- Also, in ABAP, CDS Simple Types can be used after the expression ‘TYPE’ to define the type of a variable.
DATA cds_simple_type TYPE ZPASTRY_CUSTOMER_ADDRESS.
cds_simple_type = '370 rue Léon Gambetta'.
2. CDS Enumerated Types
CDS Enumerated Types are used to limit and control the values transmitted to CDS Elements or CDS Paramters.
- CDS Enumerated Types are used to type the elements or parameters of a CDS.
Let’s create an Enumerated Types CDS, which lists the different types of preparation allowed by the bakery:
@EndUserText.label: 'Preparation type'
@EndUserText.quickInfo: 'Preparation type of the product'
@EndUserText.heading: 'Preparation type'
define type ZPREPARATION_TYPE: abap.int1 enum
{
@EndUserText.label: 'Pastry'
PASTRY = initial;
@EndUserText.label: 'Bakery'
BAKERY = 1;
@EndUserText.label: 'Sandwich'
SANDWICH = 2;
}
Then we add it to the ZI_PASTRY CDS, which defines the products available in the bakery.
@ClientHandling.type: #CLIENT_DEPENDENT
@AbapCatalog.deliveryClass: #APPLICATION_DATA
@AccessControl.authorizationCheck: #NOT_REQUIRED
@EndUserText.label: 'Pastry'
define table entity ZI_PASTRY
{
key uuid : uuid;
description : abap.char( 50 );
type : ZPREPARATION_TYPE;
@Semantics.amount.currencyCode: 'currency'
unit_amount : abap.curr( 6, 2 );
currency : abap.cuky( 5 );
//A pastry can be linked to many orders (so many orders items) or may not be in any order
_ordersItems : association of one to many ZI_BAKERY_ORDERS_ITEMS on $projection.uuid = _ordersItems.pastryid;
}
- In ABAP, a CDS Enumerated Type can be used directly for variable typing.
DATA cds_enum_type TYPE ZPREPARATION_TYPE.
cds_enum_type = ZPREPARATION_TYPE-bakery.
Conclusion
In this article, we look at new techniques for typing CDS Elements or Parameters. And that they can also be used directly in ABAP.