If you want to call one of your APIs locally for testing purposes, or to use it in one of your development objects, you can use a local proxy ( On premise or Private Cloud only ).
For example, let’s take the following API ( API_OUTBOUND_DELIVERY_SRV ) on S/4 Hana Private Cloud: https://api.sap.com/api/OP_API_OUTBOUND_DELIVERY_SRV_0002/overview
We’ll use this API to update an outbound delivery in SAP.
Developing and calling the API
To call the API in local development, proceed as follows:
- Publish service ( here oData V2 )
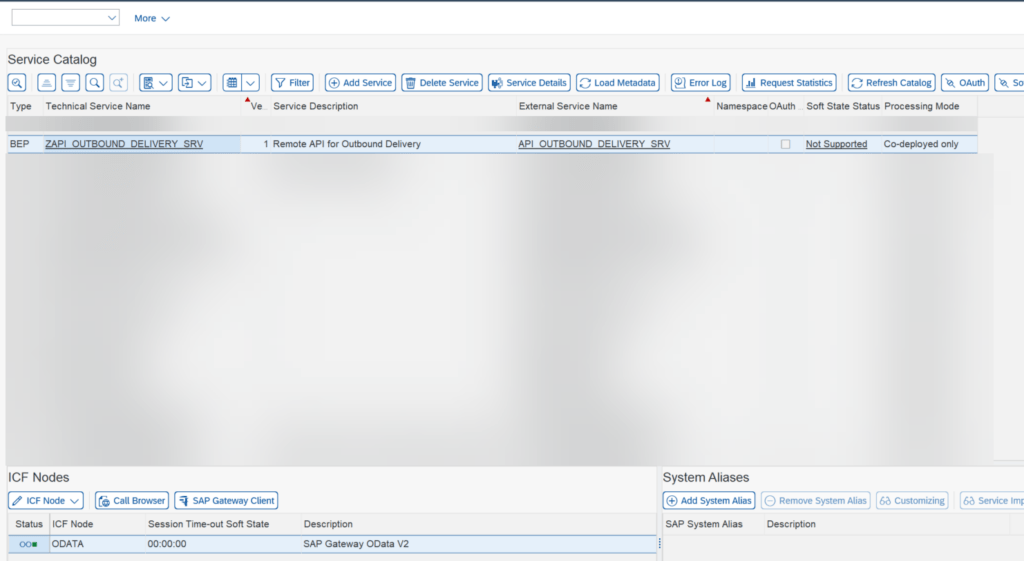
- Check for ETag by looking in the SEGW project: here DeliveryVersion (to be used later)
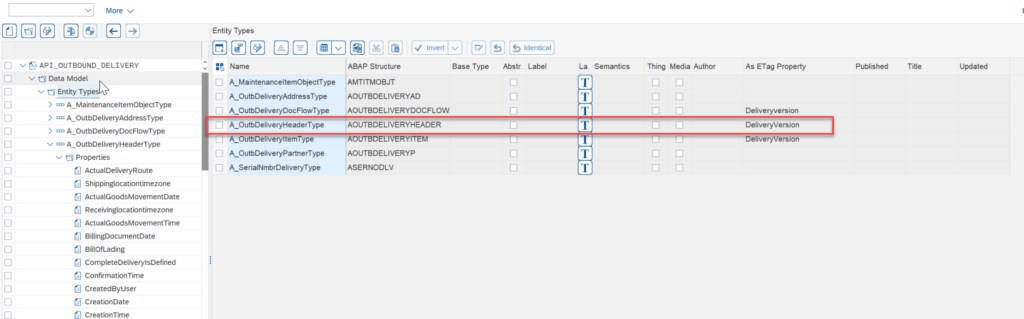
Finally, we write the code
1/ Declaring variables and creating the client proxy
* GET
DATA: lo_client_proxy TYPE REF TO /iwbep/if_cp_client_proxy,
lo_read_request TYPE REF TO /iwbep/if_cp_request_read_list,
lo_resource TYPE REF TO /iwbep/if_cp_resource_entity,
lo_response TYPE REF TO /iwbep/if_cp_response_read,
lo_read_response TYPE REF TO /iwbep/if_cp_response_read_lst,
ls_entity_key TYPE cl_api_outbound_del_02_mpc=>ts_a_outbdeliveryheadertype,
ls_business_data TYPE cl_api_outbound_del_02_mpc=>ts_a_outbdeliveryheadertype.
* Création du Proxy client
lo_client_proxy = /iwbep/cl_cp_client_proxy_fact=>create_v2_local_proxy( VALUE #( service_id = 'API_OUTBOUND_DELIVERY_SRV' service_version = '0001' ) ).
2/ Creating the GET request to obtain the ETag
lo_read_request = lo_client_proxy->create_resource_for_entity_set( 'A_OutbDeliveryHeader' )->create_request_for_read( ).
lo_read_response = lo_read_request->execute( ).
ls_entity_key = VALUE #(
deliverydocument = iv_deliverynumber ). "Delivery to update
lo_resource = lo_client_proxy->create_resource_for_entity_set( 'A_OutbDeliveryHeader' )->navigate_with_key( ls_entity_key ).
" Execute the request and retrieve the business data
lo_response = lo_resource->create_request_for_read( )->execute( ).
lo_response->get_business_data( IMPORTING es_business_data = ls_business_data ).
Data received from the API is placed in ls_business_data
3/ Create PATCH request to modify DeliveryDocumentBySupplier field
" Navigate to the resource and create a request for the update operation
DATA: lo_patch_resource TYPE REF TO /iwbep/if_cp_resource_entity,
lo_patch_request TYPE REF TO /iwbep/if_cp_request_update,
ls_patch_data TYPE cl_api_outbound_del_02_mpc=>ts_a_outbdeliveryheadertype,
lo_patch_response TYPE REF TO /iwbep/if_cp_response_update.
lo_patch_resource = lo_client_proxy->create_resource_for_entity_set( 'A_OutbDeliveryHeader' )->navigate_with_key( ls_entity_key ).
lo_patch_request = lo_patch_resource->create_request_for_update( /iwbep/if_cp_request_update=>gcs_update_semantic-patch ).
" ETag is needed
lo_patch_request->set_if_match( CONV string( ls_business_data-deliveryversion ) ).
ls_patch_data = VALUE #(
DeliveryDocumentBySupplier = iv_lifex "External ID to update
deliverydocument = iv_deliverynumber "In OData V2, it's needed to add also the key in the body request).
" Execute the request and retrieve the business data
lo_patch_request->set_business_data( is_business_data = ls_patch_data
it_provided_property = VALUE #( ( |DELIVERYDOCUMENTBYSUPPLIER| ) ) ).
lo_patch_response = lo_patch_request->execute( ).
Conclusion
Here’s a solution for calling APIs internally within an ABAP program. With this method, there is no HTTP header specified in the request, since everything is done internally in the same SAP system.
However, the /IWBEP/CL_CP_CLIENT_PROXY_FACT class is not available on the cloud (BTP, ABAP environment and S/4 Cloud). When developing in these environments, it’s best to use APIs that have been created using the RAP framework, and thus use EML to interact directly with the business object, or use the /IWBEP/CL_CP_FACTORY_REMOTE class instead, and thus create a remote proxy.
For unit tests, you can use the /IWBEP/CL_CP_FACTORY_UNIT_TST class in ABAP Cloud.